Table of Contents
If you are a frontend developer, surely you have already spent hours upon hours watching tutorials, completing courses, and coding apps. Do you sometimes feel stuck? We are here to help you! We asked our devs for their best React tips to help those who want to go from beginner to master.
Our frontend team is always eager to talk about React since it’s our main frontend stack of choice. Adding to that, the developers offer different perspectives – some more technical, some more related to the learning approach.
You might also be interested:
- Devs Talk About 4 Popular Sites That Use React JS
- 8 React Front-End Developer Interview Questions (With Answers)
Without further ado, here are some of our top React tips!
Top React Tips From React Developers
1. Learn TypeScript Early On
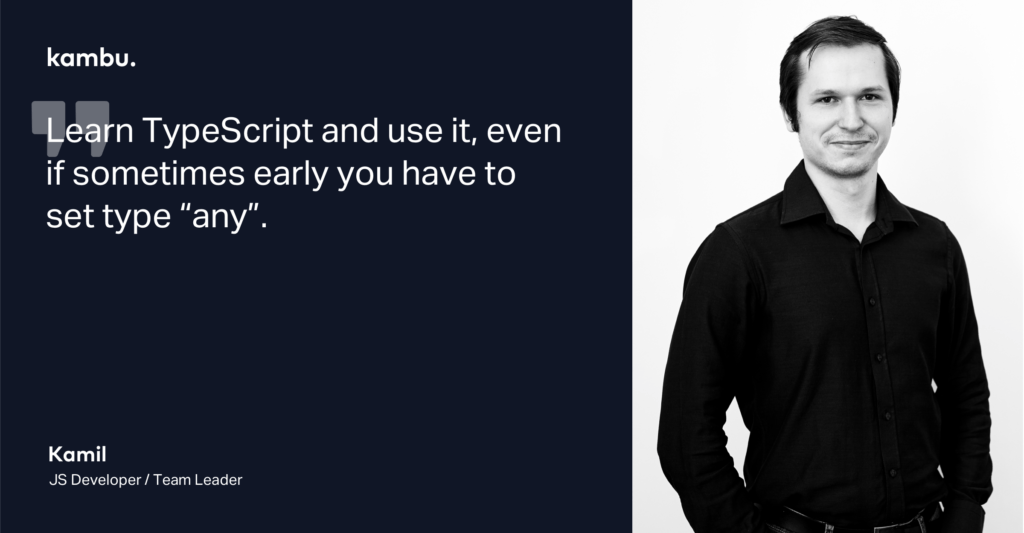
Learn TypeScript and use it, even if sometimes early you have to set type “any”.
If you are not familiar with TypeScript, the first steps can be confusing and you could ask yourself, “Do I really need it?”. If you do not know too much about TS, probably you cannot type everything correctly and you sometimes type “any”, but with time, you realize how helpful TS is.
Today, TS can be easily implemented in React projects. When using create-react-app, you need to add a flag and that’s it: you have all you need to write in TypeScript. There are many cheat sheets on the internet, which helps typing something, especially when you are using TS with React. One example: GitHub – Cheatsheets for experienced React developers getting started with TypeScript.
TypeScript Example
Let’s consider the following code. Imagine you have a custom tooltip in your application and you use them in X places. You have the correct type and you have three required properties. If you are writing only JavaScript code, you may not notice that you are not passing core property and your application throws an error because you expect e.g. item object and you are trying to get property, but actually, you have undefined instead of the desired property.
type TTooltipProps = {
item: TMap;
title: string;
key: number;
}
const Tooltip: React.FC<TTooltipProps> = ({
item, key, title,
}) => (
// Some magic here
<div>...</div>
);
But remember, TypeScript is not only about types!
There are a lot of other useful features like Enums, Generics, Abstract Classes, Decorators, and more. If you want to be an advanced developer, you have to learn how to use these cool things and how they can help improve your work and code.
– Kamil
2. Don’t Forget to Remove the Listener When Unmounting Components!
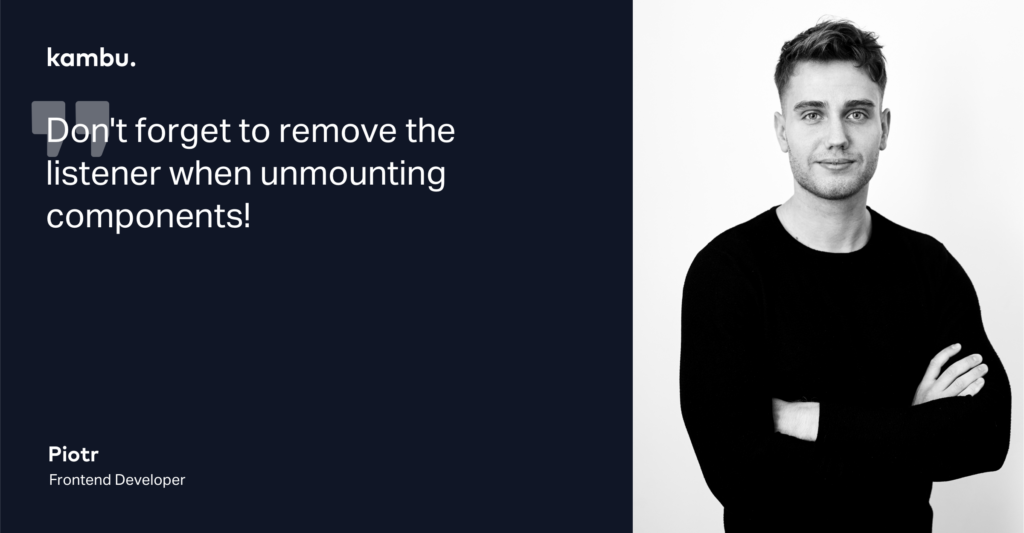
Event listeners, such as Keyboard Listeners, Event Listeners, etc, are important methods in React. When you add listeners on useEffect like onMouseOver, onScroll, onClick, etc., it will create listeners for you, but not everyone knows how important it is to remove it.
Otherwise, when you back to the component, it will create another listener object which contributes to memory leaks and unexpected listeners behavior.
So the best solution is to remove listeners, freeing out memory and providing you with uninterrupted behavior.
Removing Listener Example
useEffect(() => {
window.addEventListener("scroll", handleKeyUp);
return () => {
window.removeEventListener("scroll", handleKeyUp);
}
}, []);
– Piotr
3. Functional Components Tend To Be More Useful Than Class Components
Functional components are much lighter than class components because there is a bunch of functionality inside the class components, whether we need it or not.
For functional components, we have the option of adding in functionality if it is required. Plus, functional components, since they are pure functions (if they depend only on props, without the logic inside), are much easier to test.
Of course, there are some cases when class components may be more useful, but the React team seems to focus more on the functional components and hooks.
– Jakub
4. Avoid Duplications by Making Your Code More Reusable!
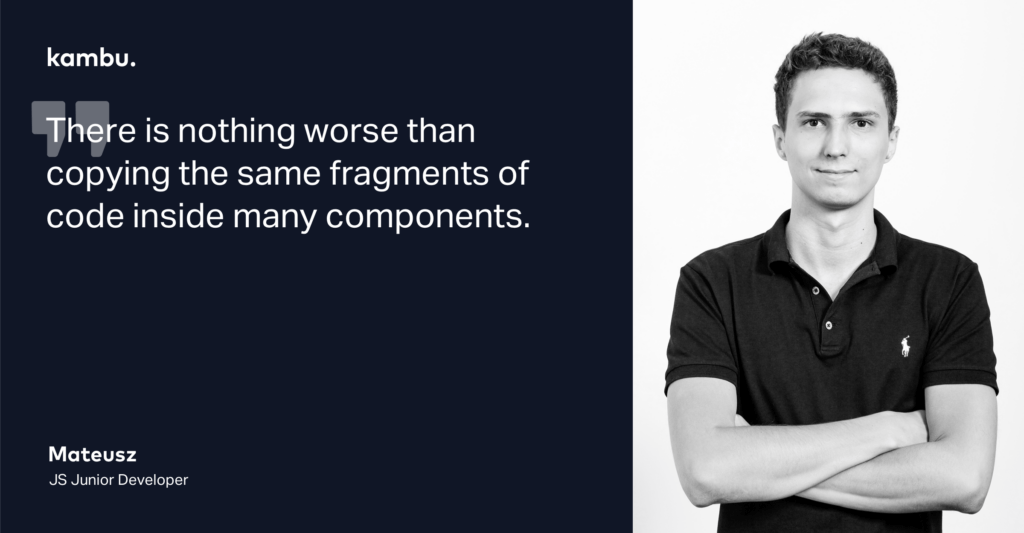
One of the key traits of an advanced developer is thinking globally about the project and its maintenance. When you are writing functionality for a specific component you should consider the fact that maybe there are (or there would be) other places in the system that have similar functionality.
There is nothing worse than copying the same fragments of code inside many components.
The best way to apply the above-mentioned rule on a daily basis is using react functional components to have the benefits of using and creating your own hooks! This gives us the possibility of sharing the same logic between react components, which makes your code more readable, reusable, and clean.
Example Project
Let’s consider the example of a project that has the following rule: every time we want to edit a list item we need to open the form on the right side of the view.
To achieve the desired effect, we have to add some specific class name to the body of the document to change the layout. Also after closing the form we need to clean the effect.
When you use class components, your solution will look like the example below:
class EditForm extends React.Component {
componentDidMount() {
document.querySelector('body').classList.add('edit-side-form');
}
componentWillUnmount() {
document.querySelector('body').classList.remove('edit-side-form');
}
render() {
return <div>Your form here.</div>;
}
}
The logic mentioned above should be included in every component which requires it – that generates the code duplication problem. So now, let’s create our own custom hook useStylesOnMount.js to handle it!
import { useEffect } from 'react';
export default (className) => {
useEffect(() => {
document.querySelector('body').classList.add(className);
return () => {
document.querySelector('body').classList.remove(className);
};
}, [className]);
};
Solution based on our custom hook:
export const EditForm = () => {
useStylesOnMount('edit-side-form');
return <div>Your form here.</div>;
};
We achieve the same effect using one line of code inside the EditForm component and that’s not all! Now by using this one-liner we have the possibility to add different class names to the body element passing the className argument to our hook function.
The problem from the example is trivial, but consider that you have to make something more complicated inside many places in your app – then just remember about this rule before you start using the copy-paste method!
– Mateusz
5. Write Unit Tests For Methods or Component Rendering
Tests are important, but a lot of developers don’t like to write them.
If you are using redux for state management with redux-thunk, sometimes you have more lines of code in actions and this is critical functionality, so it would be nice to have the correct tests. Why? When you have to refactor your code, tests will tell you if you broke something. When you have to change something, firstly change your test and then change your implementation. Then you can be sure that nothing will break after the changes.
Also important are tests of your components. To begin with, all you need to do is make an abstract test for component rendering.
Test Example
Let’s consider the code below:
import React from 'react';
import { mount } from 'enzyme';
import { Provider } from 'react-redux';
import { MemoryRouter } from 'react-router-dom';
export default ({
Component,
componentName,
props = {},
storeConfig = {},
}) => {
it(`should render <${componentName} /> Component without throwing an Error`, () => {
const renderTest = () => mount(
<Provider store={storeConfig}>
<MemoryRouter>
<Component {...props} />
</MemoryRouter>
</Provider>,
);
expect(renderTest).to.not.throw(Error);
});
};
This is a simple test, but you can use it to test your functional component like this:
describe('<LoginPage />', () => {
basicFnComponentTest({
Component: LoginPage,
componentName: 'LoginPage',
storeConfig: {
page: {
login: {
form: {},
recoverPassword: {},
},
},
session: { waitingForResponse: true },
version: {
downloadLinks: [],
downloadDocuments: [],
},
},
props: {}
});
});
Pretty easy, but you know that your component does not throw an error after your changes.
– Kamil
6. Share your Knowledge and Experience
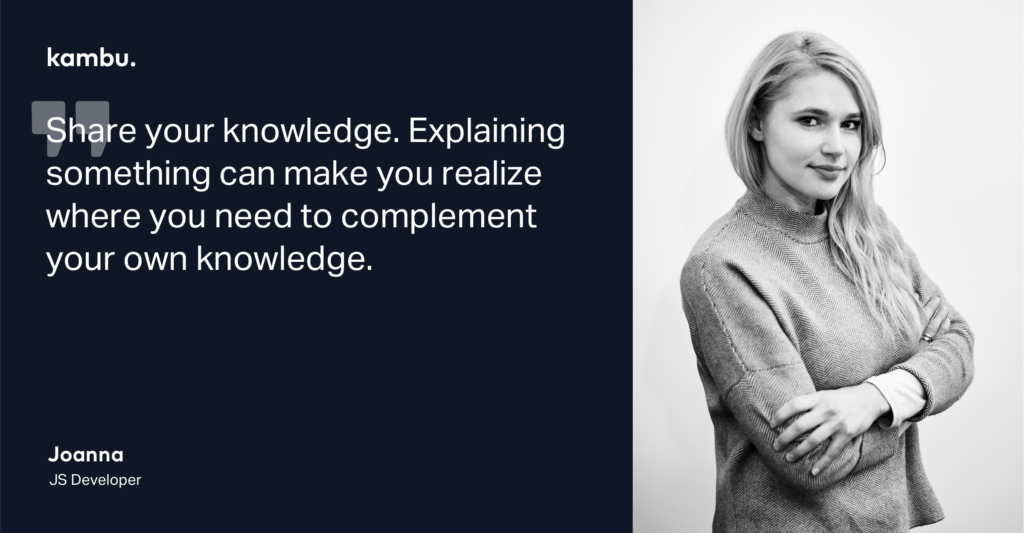
Share your knowledge and experience. The process of explaining something can make you realize where you need to complement your own knowledge.
– Joanna
7. Always Think of Multiple Solutions
Before any implementation, try to find more than one way to implement a solution. It often reveals some issues that otherwise you would notice only when implementing.
A useful practice is to discuss implementation plans with someone or work using a TDD (test-driven development) process.
– Joanna
8. Ask Questions to Learn From Others
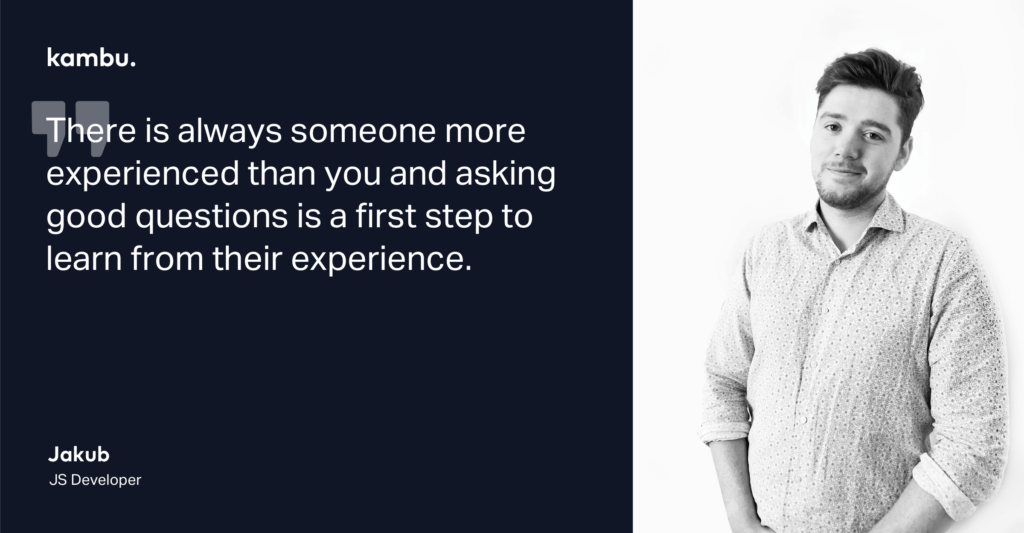
Remember that there is always someone more experienced than you, and asking good questions is a first step to learn from those people’s experiences.
Especially at the beginning of your journey with programming, there will be many moments when you’ll get stuck with something and deal with thousands of thoughts per minute, but no solution.
If you don’t solve the problem by yourself in a short period of time, there is no point to invent the wheel once again. Just ask someone for help and take the most from it.
– Jakub